ELisp: Symbol vs String
In lisp, do not use symbol when you can use string, because it pollutes symbol table.
Which of the following should you use?
(search-forward "cat" nil "NOERROR" ) (search-forward "cat" nil 'NOERROR )
Using symbol is problematic. It adds the symbol to the symbol lookup table obarray , and may already have a value, possibly of nil.
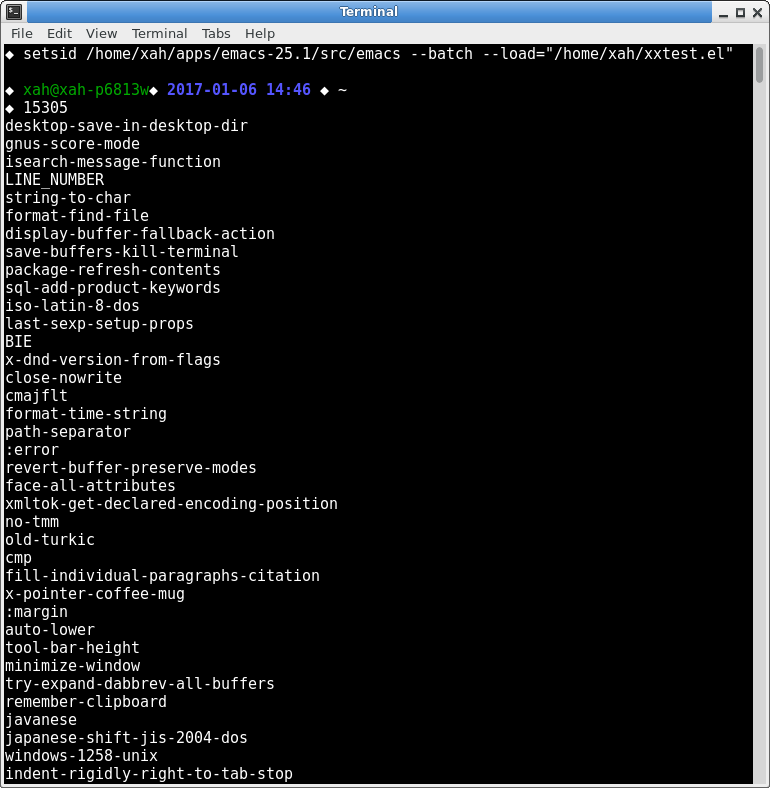
Here's explanation.
In elisp:
- There's no named parameters. It's just ordered parameters.
- Also, there is no default argument mechanism.
- When a parameter doesn't receive argument, its value is nil.
- There's no way to tell if a parameter isn't passed a argument or if it was passed nil.
[see ELisp: Function Optional Parameters]
So, this create a situation. When the parameters are meant to be true or false, you have code like this:
(f t nil t t nil nil)
, which is hard to understand.
For example, the search-forward
function:
(search-forward STRING &optional BOUND NOERROR COUNT)
and you may call it like this:
(search-forward "cat" nil t )
which means, search for βcatβ, to the end of buffer (not bounded), and do not report error if not found (and move cursor to the found text or end of search limit (e.g. end of buffer) if none found.).
One way to make it more readable is to replace some t value by a string, since string in elisp is also non-nil. (Warning: some function's parameter differentiate t and other non-nil values, so you can't always just pass it a string without consideration.
For example, search-forward
's 3rd parameter and
replace-match
's 4th parameter.
)
You can make a non-nil value more readable by passing a string instead, like this:
(search-forward "cat" nil "NOERROR" )
A couple years ago (~2015) a elisp hacker suggested to me to replace string
"NOERROR"
by a symbol
'NOERROR
. The reason given was that it costs more to create a string than symbol.
I didn't really agree, because:
- If the cost of creating string is costy to the degree that programers have to think about, then the language would be a very impractical one. Doesn't matter how emacs implement string, this shouldn't matter, because strings are used in just about every function, including the doc string.
- Using symbol as non-nil is not intuitive. Because symbol is not in other languages, less well-understood to programers.
However, i sometimes went along, just to experiment. So, in some parts of my code, you'll see 'NOERROR
and in other parts you see "NOERROR"
.
Today, i'm changing all of them back to the string form "NOERROR"
.
- Because, using symbol pollutes the symbol table. That it, it creates the symbol and insert into obarray. Creating Symbols (ELISP Manual)
- The symbol may already have a value, possibly nil. This would cause incorrect program.
Here's the functions that changed.
- Emacs: Quote Lines
- Emacs: Change to Title Case π
- Emacs: Escape Quotes Command π
- Emacs: Delete Text Block
- Emacs: Cycle Space Hyphen Underscore
- Emacs: Reformat Lines for Source Code
- Emacs: Change Brackets/Quotes
- ELisp: HTML image-link Command
Emacs Lisp Misc Essays
- Text Processing vs Structured
- Elisp coding style: let forms
- ELisp Naming Convention
- Some and Every
- What is the Function fn?
- Symbol vs String
- Meaning of Lisp List, Function Type, and Syntax Coloring
- Elisp vs Perl: Validate File Links
- Emacs: Relation of Curor Position to Begin/End of Matching Pairs in Source Code
- Text Processing: ELisp vs Perl
- Can ELisp Fix Its Namespace Problem by Copying JavaScript Practice?
- ELisp vs JavaScript
- Controversy of Common Lisp Package in ELisp
- Lisp List Problem
- Lisp-1 vs Lisp-2
- ELisp Problems: Trim String, Regex Match Data, Lacking Namespace
- Functional Programing: Function Output Should Always Have the Same Structure