Xah Talk Show 2022-09-07 Emacs Lisp for Beginner
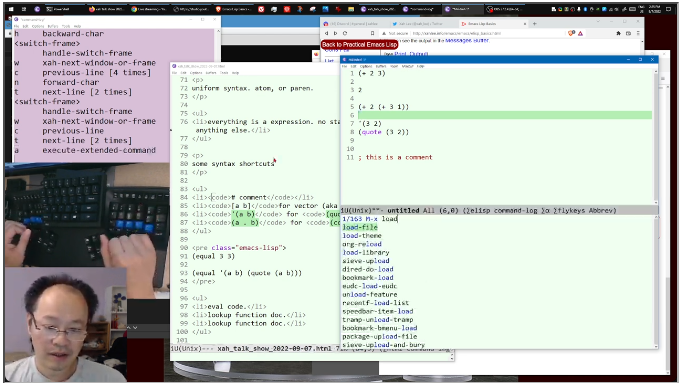
- https://youtu.be/sDKUKDdtspQ
- Xah Talk Show 2022-09-07 Emacs Lisp for Beginner
- 0:00 Intro
- 1:50 Background on Emacs lisp
- 15:07 Emacs Fundamentals
- 23:37 How to Evaluate code
- 25:45 Intro Overview
- 27:07 How to Print
- 30:25 Strings
- 35:01 String Functions
- 37:13 Substrings
- 40:53 Format String
- 42:11 Arithmetic
- 43:15 Convert Int/Float/String
- 44:32 Lisp Types
- 49:00 Boolean
- 54:00 Test Equality
- 56:02 Variables
- 58:36 Block of Expressions
- 1:02:37 Lexical Scope vs Dynamic Scope
- 1:13:08 Loops
- 1:15:43 Lists
- 1:21:41 Define Functions
- 1:23:15 Overview
- 1:27:37 Questions
- assume you have used emacs for a month or two.
- assume you have some familiarity with a programing language. e.g. python php ruby JavaScript java golang
2 parts to emacs lisp learning.
- Emacs lisp items common to every programing language. e.g. string, variable, function, boolean, flow control, loop, datatypes, input/output.
- Emacs specific emacs lisp, such as text manipulation, cursor, buffer, major/minor mode, syntax coloring, gui window/frame, etc.
for Emacs specific emacs lisp, there are 2 parts:
- text processing. e.g. write command to manipulate text, insert, delete, move, refactor code, template, etc.
- emacs system. e.g. font, syntax coloring, minor mode, major mode, manipulate window/frame size, write a dir viewer, pdf viewer, image viewer, chat interface, email client, etc.
- everything is a expression. No statements.
- uniform syntax. Either atom, or paren.
some syntax shortcuts
; comment
[a b]
for unevaluated vector (aka tuple)'(a b)
for(quote (a b))
(a . b)
for(cons a b)
(equal 3 3) (equal '(a b) (quote (a b)))
- eval code.
- lookup function doc.
go thru the tutorial
lexical scope vs dynamic scope
Lexical scope, basically means, text based variable scope. Meaning, what you see is what you get. Meaning, that a variable's scope is inside a syntactic unit of the language, such as paren or curly bracket.
dynamic scope, is basically, a time based scope.
LISP, originally stand for list processor
;;; lexical-binding: t; -*- lexical-binding (+ 2 3) 2 (+ 2 (+ 3 1)) '(3 2) (quote (3 2)) ;; (f a b c) (message "hello world factorial" ) ; this is a comment (message "hello world factorial" ) (insert "abc") ;; abc (insert "something more fancy\nok?") ;; something more fancy ;; ok? (length "abc") (length "🤩") ;; python "double" ;; 'single' ;; """triple""" ;; '''triple''' ;; JavaScript template quote `t` (substring STRING FROM &optional TO) (substring "abcdefg" "1" ) (substring "abcdefg" 1 ) (substring "abcdefg" 3 ) (equal (substring "abcdefg" 3) "defg" ) (format "hello" ) (format "hello i have %s cats" 34 ) (format "%s" 34 ) (string-to-number "3") (number-to-string 3) (/ 7 2) (/ 7 2.0) (eq nil (list)) (eq nil '() ) nil 'nil (eq nil 'nil) (or nil 3) (eq t 't ) (if t "yes" "no") (if 0 "yes" "no") (if "" "yes" "no") (if [] "yes" "no") ;; variable (setq x 3) ;; x (equal x 3) (let (x) (setq x 4) (message "%s" x)) (if (> x 2) (message "yes, bigger than 2") (insert "wow") (message "no. it is smaller than 2")) (if (> x 2) (progn (message "yes, bigger than 2") (insert "wow")) (message "no. it is smaller than 2")) ;; loop (while (< x 9) (message "%s" x) (setq x (+ x 1))) (list 3 4 5) (list 3 (list 3 4 5) 5) (length (list 3 4 5)) (append (list 3 4 5) (list "a" "b")) (equal (list 3 (list 3 4 5) 5) (list 3 (list 3 4 5) 5)) (mapcar (lambda (x) (+ x 1)) (list 3 4 5)) (defun ff (x) "add 2 to x" (let () (+ x 2))) (ff 3)
byte compile emacs lisp