JS: Semicolon
JavaScript syntax is very complex, such that semicolon can sometimes be omitted, sometimes not, and there is no simple rule for programer to remember when it can be omitted.
Rule for Always Using Semicolon Style
- ALWAYS add semicolon at end of assignment.
- A redundant semicolon is a empty statement. Extra semicolon won't hurt.
need semicolon:
- Assignment needs semicolon
let x = 3;
- Sequence of expressions, such as function call, need semicolon to separate them. e.g.
f(3); f(4);
.
no need semicolon:
function f (x) {return x+1;}
.for
while
if
switch
try
Rule for Omitting Semicolon Style
Do not add semicolon, except:
- A line should never start with left parenthesis () nor left square bracket [].
- If a line starts with parenthesis or square bracket, add a semicolon in the beginning of line (or end of previous line).
Advantage of Omitting Semicolon
- Less typing.
Disadvantage of Omitting Semicolon
- Code becomes layout dependent. If you join 2 lines into 1 line, the code won't run correctly.
Use Automatic Formatting Tool
Use Deno to autoformat code.
deno adds semicolon automatically.
When Do You Need Semicolon by JavaScript Grammar?
The full spec is fairly complex.
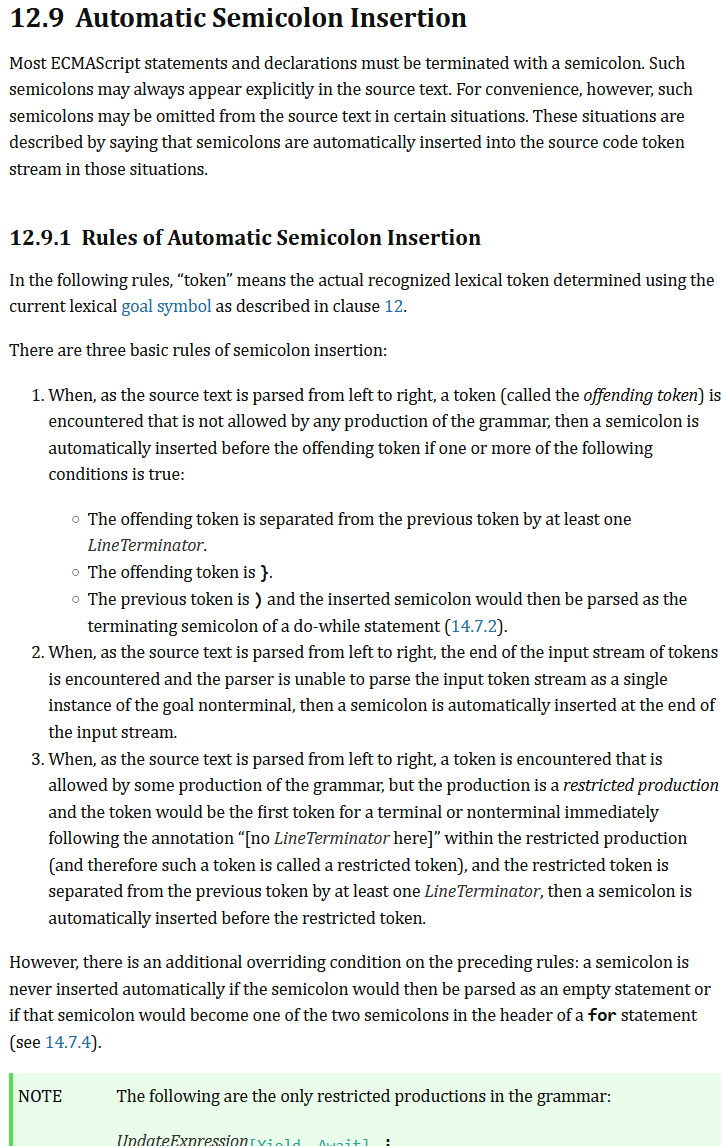
Tips on Semicolon
Return Statement Must be in One-Line
function ff() { // bad return [3, 4, 5]; } console.log(ff() === undefined);
Example of Bad Omitted Semicolon
/* example of erro due to semicolon omission. you intended to assign the first function, and eval the second. but js compiler tried to feed the second function as arg to your first function */ const f = function () { return 3; } // bad semicolon omission (function () { return 4; })(); /* error: Uncaught TypeError: (intermediate value)(...) is not a function })(); ^ */